Write a Python program that takes a list of numbers as input and returns the sum of all even numbers in the list
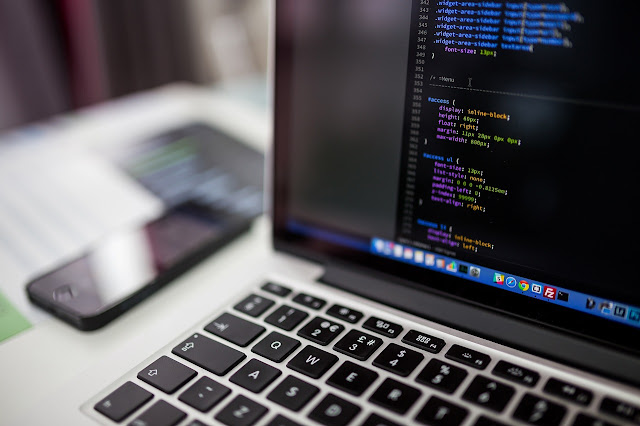
Program Code: Program Explanation: The function sum_even_numbers takes a single argument numbers, which is a list of integers. The function uses a list comprehension to create a new list of even_numbers containing only the even numbers from the input list. This is done using the modulo operator %, which returns the remainder of dividing a number by 2. If the remainder is 0, then the number is even, and it is added to the even_numbers list. The sum function is then called on the even_numbers list to compute the sum of all the even numbers in the list. The sum of the even numbers is returned as the output of the function. Overall, this function is a simple and efficient way to compute the sum of even numbers in a list of integers.