Age and Gender Checking Program in C-language
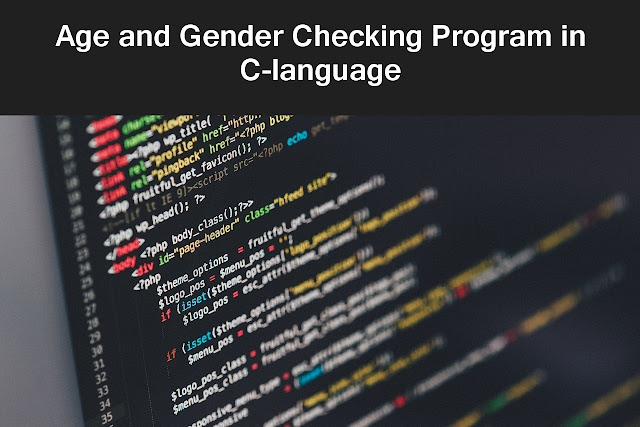
#include<stdio.h> void main() { char gen; //decleration of variable int age; //decleration of variable printf("Enter Your Gender, M for Male and F for Female"); scanf("%c",& gen); printf("Enter Your Age"); scanf("%i",& age); if(age > 16 && gen == 'F' || age > 16 && gen == 'f' ) { printf("Female is genius"); } else if(age > 16 && gen == 'M' || age > 16 && gen == 'm' ) { printf("Male have to do more effort"); } }